Java Hangman Game
This project is a Java implementation of the classic Hangman game. Players can guess letters to uncover a hidden word. The game includes features such as displaying the current state of the word, tracking guessed letters, and limiting the number of incorrect guesses.
Java Code Snippet
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Scanner;
public class HangmanGame {
private static final String FILE_PATH = "states.txt";
private ArrayList words;
private char[] word;
private boolean[] wordMask;
private char[] guessedLetters;
private int incorrectAttempts;
public HangmanGame() {
words = new ArrayList<>();
word = null;
wordMask = null;
guessedLetters = new char[26];
incorrectAttempts = 0;
}
private void readWordsFromFile() {
try (BufferedReader reader = new BufferedReader(new FileReader(FILE_PATH))) {
String line;
while ((line = reader.readLine()) != null) {
words.add(line.toLowerCase());
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void chooseRandomWord() {
Random random = new Random();
int randomIndex = random.nextInt(words.size());
String chosenWord = words.get(randomIndex);
word = chosenWord.toCharArray();
wordMask = new boolean[word.length];
}
private void printWord() {
for (int i = 0; i < word.length; i++) {
if (wordMask[i]) {
System.out.print(word[i] + " ");
} else {
System.out.print("_ ");
}
}
System.out.println();
}
private void printHangman() {
int remainingAttempts = 6 - incorrectAttempts;
System.out.println("Incorrect Attempts: " + incorrectAttempts);
System.out.println(" __________");
System.out.println(" | |");
switch (remainingAttempts) {
case 0:
System.out.println(" | |");
System.out.println(" | _/_\\ ");
System.out.println(" | |_| ");
System.out.println(" | / \\ ");
System.out.println(" | / \\ ");
break;
case 1:
System.out.println(" | |");
System.out.println(" | _/_\\ ");
System.out.println(" | |_| ");
System.out.println(" | / ");
System.out.println(" | / ");
break;
case 2:
System.out.println(" | |");
System.out.println(" | _/_\\ ");
System.out.println(" | |_| ");
System.out.println(" | ");
System.out.println(" | ");
break;
case 3:
System.out.println(" | |");
System.out.println(" | _/_ ");
System.out.println(" | |_| ");
System.out.println(" | ");
System.out.println(" | ");
break;
case 4:
System.out.println(" | |");
System.out.println(" | _/ ");
System.out.println(" | |_| ");
System.out.println(" | ");
System.out.println(" | ");
break;
case 5:
System.out.println(" | |");
System.out.println(" | |");
System.out.println(" | /| ");
System.out.println(" | |_| ");
System.out.println(" | ");
break;
case 6:
System.out.println(" | |");
System.out.println(" | |");
System.out.println(" | /|\\");
System.out.println(" | |_| ");
System.out.println(" | ");
break;
}
System.out.println(" | ");
System.out.println(" |____________|");
}
private void printGuessedLetters() {
System.out.print("Guessed Letters: ");
for (char letter : guessedLetters) {
if (letter != 0) {
System.out.print(letter + " ");
}
}
System.out.println();
}
private boolean isGameOver() {
return incorrectAttempts >= 6 || isWordGuessed();
}
private boolean isWordGuessed() {
for (boolean isLetterGuessed : wordMask) {
if (!isLetterGuessed) {
return false;
}
}
return true;
}
public void play() {
readWordsFromFile();
chooseRandomWord();
Scanner scanner = new Scanner(System.in);
while (!isGameOver()) {
System.out.println("Hangman Game");
printWord();
printHangman();
printGuessedLetters();
System.out.print("Guess a letter: ");
char guess = scanner.next().toLowerCase().charAt(0);
guessedLetters[guess - 'a'] = guess;
boolean correctGuess = false;
for (int i = 0; i < word.length; i++) {
if (word[i] == guess) {
wordMask[i] = true;
correctGuess = true;
}
}
if (!correctGuess) {
incorrectAttempts++;
}
}
if (isWordGuessed()) {
System.out.println("Congratulations! You guessed the word: " + new String(word));
} else {
System.out.println("Game over! The word was: " + new String(word));
}
}
public static void main(String[] args) {
HangmanGame hangmanGame = new HangmanGame();
hangmanGame.play();
}
}
Project Images
Sequence Diagram
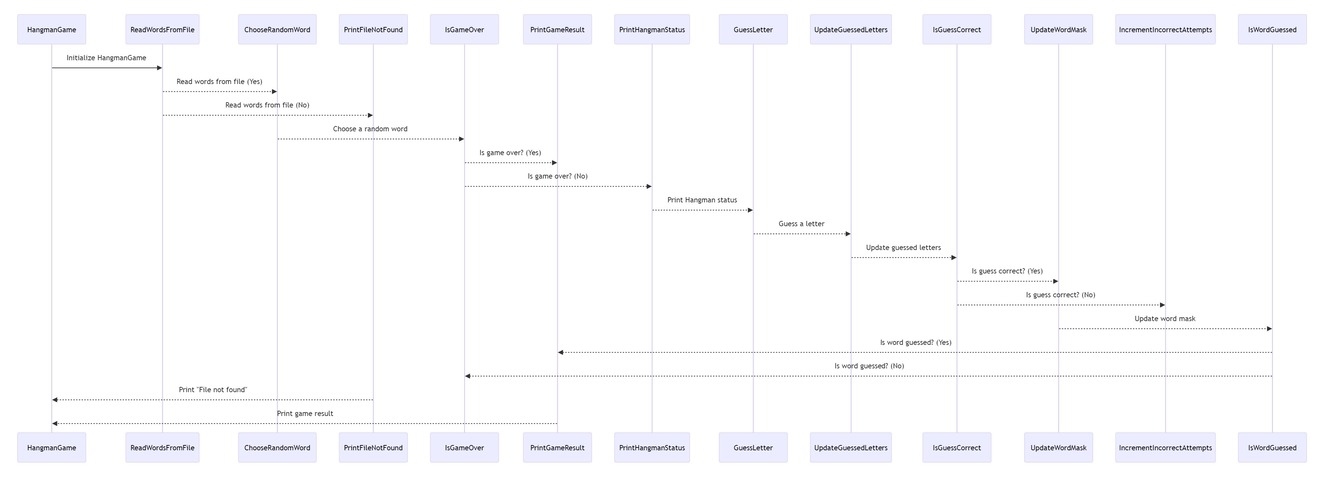
Output Image
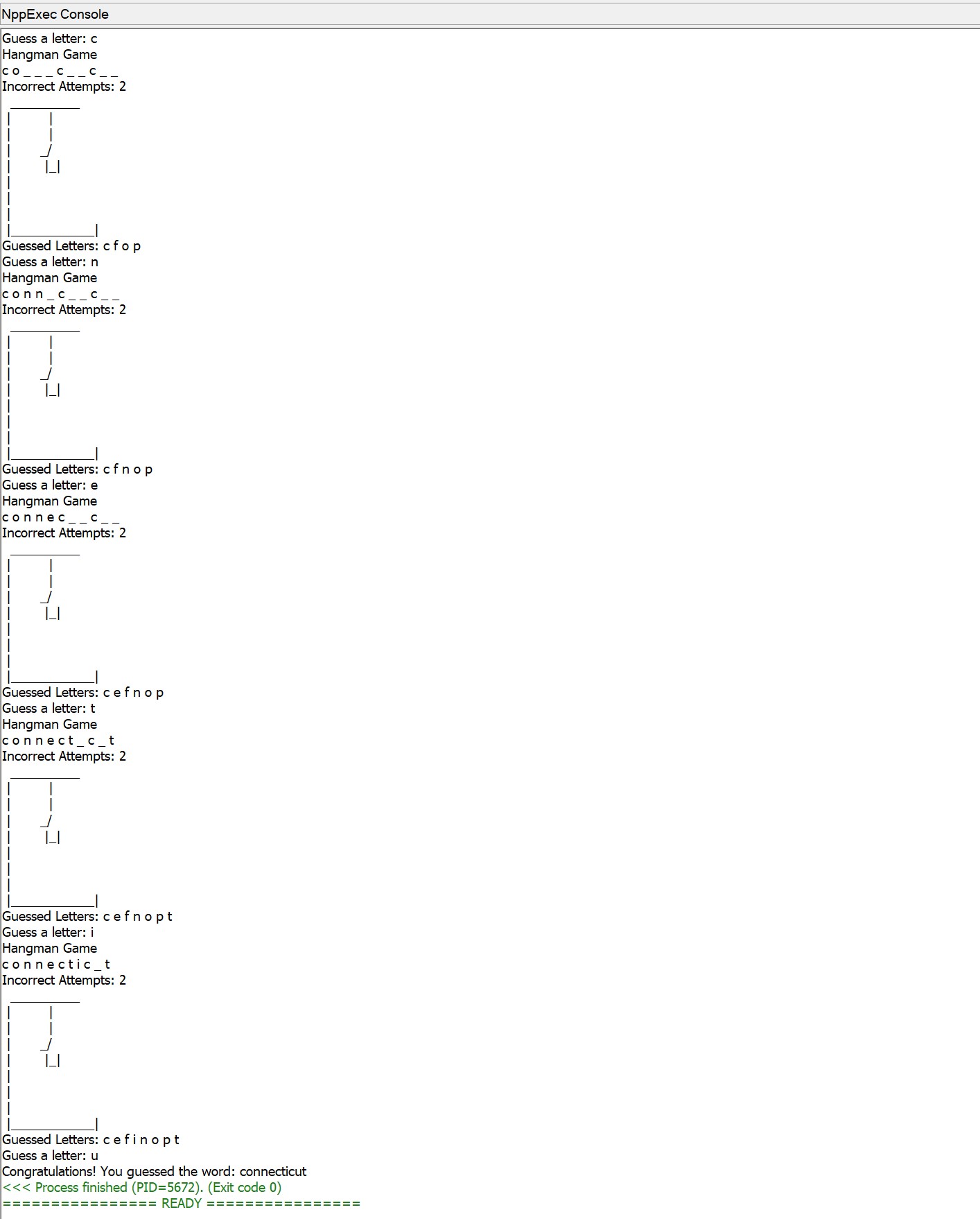
For the hangmang text document you can Download here.